Road Speed Limits by Countries
Contents
25. Road Speed Limits by Countries#
We use the data from wikipedia article:
https://en.wikipedia.org/wiki/Speed_limits_by_country
import re
import pandas as pd
import numpy as np
25.1. Get data#
wiki_link = "https://en.wikipedia.org/wiki/Speed_limits_by_country"
df = pd.read_html(wiki_link)
df = df[1]
df.head()
Country | Within towns | Controlled-access highway/ motorway/ expressway | Outside built-up areas/regional highways | Automobiles & motorcycles | Lorries or automobiles with trailer | Enforcement tolerance | |
---|---|---|---|---|---|---|---|
0 | Albania[4][5] | 40 | 110 | 80 | 80–90 | 60–70 | NaN |
1 | Argentina | 40–60 | 100–130 | 80 | 80–110 | 80 | NaN |
2 | Australia[6] | 40–60 | 100–130[fn 2] | 100[fn 3] | 100–110[fn 2] | 100[fn 3] | 3 in Victoria, 10% over speed limit in other s... |
3 | Austria[7] | 50 | 130 | 80–100 | 100 | 80–100 | NaN |
4 | Azerbaijan[8] | 40–60 | 110 | NaN | 90 | NaN | NaN |
df.columns
Index(['Country', 'Within towns',
'Controlled-access highway/ motorway/ expressway',
'Outside built-up areas/regional highways', 'Automobiles & motorcycles',
'Lorries or automobiles with trailer', 'Enforcement tolerance'],
dtype='object')
rename_cols = {
'Country': 'country',
'Trucks, or automobiles with trailer': 'trucks_and_trailers',
'Trucks, or automobiles with trailer, outside built-up areas/highways': 'trucks_and_trailers_non_built_up'
}
df.rename(columns=rename_cols, inplace=True)
df_trucks = df[rename_cols.values()]
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
Cell In[9], line 1
----> 1 df_trucks = df[rename_cols.values()]
File ~/anaconda3/envs/mini-lab/lib/python3.9/site-packages/pandas/core/frame.py:3813, in DataFrame.__getitem__(self, key)
3811 if is_iterator(key):
3812 key = list(key)
-> 3813 indexer = self.columns._get_indexer_strict(key, "columns")[1]
3815 # take() does not accept boolean indexers
3816 if getattr(indexer, "dtype", None) == bool:
File ~/anaconda3/envs/mini-lab/lib/python3.9/site-packages/pandas/core/indexes/base.py:6070, in Index._get_indexer_strict(self, key, axis_name)
6067 else:
6068 keyarr, indexer, new_indexer = self._reindex_non_unique(keyarr)
-> 6070 self._raise_if_missing(keyarr, indexer, axis_name)
6072 keyarr = self.take(indexer)
6073 if isinstance(key, Index):
6074 # GH 42790 - Preserve name from an Index
File ~/anaconda3/envs/mini-lab/lib/python3.9/site-packages/pandas/core/indexes/base.py:6133, in Index._raise_if_missing(self, key, indexer, axis_name)
6130 raise KeyError(f"None of [{key}] are in the [{axis_name}]")
6132 not_found = list(ensure_index(key)[missing_mask.nonzero()[0]].unique())
-> 6133 raise KeyError(f"{not_found} not in index")
KeyError: "['trucks_and_trailers', 'trucks_and_trailers_non_built_up'] not in index"
df_trucks.sample(10)
country | trucks_and_trailers | trucks_and_trailers_non_built_up | |
---|---|---|---|
150 | Vanuatu [76] | NaN | NaN |
51 | Greenland (Denmark) | NaN | NaN |
77 | Lebanon | NaN | NaN |
155 | Zambia | 75 | 55-70 |
132 | Sudan | NaN | NaN |
69 | Jersey | NaN | 64 (40 mph) |
90 | Moldova | 70 | NaN |
24 | CanadaMain article: Speed limits in Canada | 20–120 (12-75 mph) | 30–120 130 Proposed[17] |
43 | Faroe Islands (Denmark) | NaN | NaN |
143 | Uganda [70] | NaN | NaN |
df_trucks.country.sample(20).values
array(['Vanuatu [76]', 'South Korea (Republic of Korea)',
'Albania[5][6]Main article: Speed limits in Albania',
'Hungary Main article: Speed limits in Hungary', 'Morocco', 'Niue',
'San Marino', 'Peru', 'Micronesia [48]',
'Belarus Main article: Speed limits in Belarus', 'Nicaragua',
'Palestine', 'Singapore',
'Ireland Main article: Speed limits in the Republic of Ireland',
'Georgia Main article: Speed limits in Georgia', 'Curaçao',
'Czech Republic Main article: Speed limits in the Czech Republic',
'Samoa', 'Norfolk Island', 'Finland'], dtype=object)
25.2. Clean Up Dataset#
def clean_country_name(name):
res = name.split("[")[0]
res = res.split("Main article")[0]
res = res.strip()
return res
df_trucks['country'] = df_trucks.country.apply(clean_country_name)
/Users/lei.ma/opt/anaconda3/envs/mini-code/lib/python3.6/site-packages/ipykernel_launcher.py:1: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
"""Entry point for launching an IPython kernel.
df_trucks.sample(20)
country | trucks_and_trailers | trucks_and_trailers_non_built_up | |
---|---|---|---|
122 | Serbia | 70 | 80 (car) 90/100 (truck) |
62 | Iraq | 90 | 120 |
44 | Fiji | NaN | NaN |
93 | Mongolia | NaN | NaN |
47 | Georgia | 60 | 80 |
105 | Norfolk Island | NaN | 50 |
74 | Kyrgyzstan | 70 | 90-70 |
42 | Ethiopia | NaN | NaN |
130 | Spain | 70–80[fn 32] | 80–90[fn 33] |
26 | Chile | 100 (90 for trucks) | 100 |
14 | Belgium: Wallonia Brussels | 60–90 | 90 |
27 | Christmas Island | NaN | 90 |
39 | Egypt | NaN | NaN |
77 | Lebanon | NaN | NaN |
96 | Namibia | NaN | NaN |
24 | Canada | 20–120 (12-75 mph) | 30–120 130 Proposed[17] |
38 | Ecuador | 40–70 (50–90 for buses) | 90 |
16 | Benin | NaN | NaN |
117 | Qatar | NaN | NaN |
7 | Austria | 70–100[fn 5] | 80–100[fn 6] |
df_trucks.trucks_and_trailers.values
array([nan, '60–70', '80', '90', nan, nan,
'80–100 trucks and road trains only', '70–100[fn 5]', nan, nan,
nan, nan, '70', '60–70', '60–90', nan, nan, nan, '80', '90', '80',
'70', '80', nan, '20–120 (12-75\xa0mph)', '60-80',
'100 (90 for trucks)', nan, '60', '60',
'90 (buses), 75 (heavy goods)', '80', nan, nan, '80', '80', '80',
nan, '40–70 (50–90 for buses)', nan, nan, '90', nan, nan, nan,
'80', '60[fn 14]-110', '60',
'80 (trucks) / 100 (automobiles with trailer and buses)', nan,
'80 (school buses 60)', nan, nan, nan, nan, nan, '70[fn 20]', '70',
'80', '65', '80', '70–110', '90', '80–90', nan, '80', '70', nan,
'80 (over 8t)', nan, nan, '30', nan, '70–100', '70', '90', '80',
nan, nan, '80', '70–80–90', '90', '?', nan, '100', nan, '50–70',
nan, '60', nan, '70', nan, nan, nan, nan, '95 (60\xa0mph)', nan,
nan, nan, 'regular within built-up area restrictions', nan,
'80–90', nan, nan, nan, nan, nan,
'80, 60 without brakes on trailer', '80\xa0km/h', '60', nan, nan,
'70–80',
'90 (buses)80 (for trucks)70 (for school buses and dangerous goods)',
'40–80', '70', '70–80', nan, '8090 (E-roads)', '70–90', nan, nan,
'70', '60', '90', '80', '60', '40–80', '60', '40–60',
'70–80[fn 32]', '40 (25\xa0mph) (TukTuk)', nan, 'none',
'Lorries 80 90 without trailer on motorways only (as posted). Buses 80 100 without trailer, on any road (as posted).',
'80', '60–80', '100',
'TruckBangkok Metropolitan & Pattaya City: 60Others: 80Long VehicleBangkok Metropolitan & Pattaya City: 45Others: 60',
'50', nan, '80', nan, nan, '70–90', '50–80',
'80–97 (50-60\xa0mph) dependent on class (64–97 (40-60\xa0mph) in Scotland)[fn 39]',
'Restrictions only in few states, typically 16\xa0km/h (10\xa0mph) lower',
'none', '70', nan, nan, '40–60', '70', nan, '75', '80'],
dtype=object)
def clean_speed(speed, mode=None):
if mode is None:
mode = "max"
if isinstance(speed, str):
res = re.sub('\[[^>]+\]', '', speed)
res = re.sub('\([^>]+\)', '', res)
res = [int(e) for e in re.split("[^0-9]", res) if e != '']
else:
res = None
if res:
if mode == "max":
res = max(res)
elif mode == "min":
res = min(res)
else:
raise NotImplementedError(f"mode = {mode} has not yet been implemented")
else:
res = None
return res
test_string = df_trucks.iloc[138].trucks_and_trailers
clean_speed(test_string)
80
df_trucks['trucks_and_trailers'] = df_trucks.trucks_and_trailers.apply(clean_speed)
df_trucks['trucks_and_trailers_non_built_up'] = df_trucks.trucks_and_trailers_non_built_up.apply(clean_speed)
/Users/lei.ma/opt/anaconda3/envs/mini-code/lib/python3.6/site-packages/ipykernel_launcher.py:1: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
"""Entry point for launching an IPython kernel.
/Users/lei.ma/opt/anaconda3/envs/mini-code/lib/python3.6/site-packages/ipykernel_launcher.py:2: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
df_trucks.sample(20)
country | trucks_and_trailers | trucks_and_trailers_non_built_up | |
---|---|---|---|
117 | Qatar | NaN | NaN |
153 | Vietnam | 70.0 | 70.0 |
31 | Croatia | 80.0 | 80.0 |
18 | Bosnia and Herzegovina | 80.0 | NaN |
5 | Andorra | NaN | NaN |
104 | Nigeria | NaN | NaN |
106 | North Korea | NaN | NaN |
97 | Micronesia | NaN | NaN |
111 | Papua New Guinea | NaN | NaN |
61 | Iran | 110.0 | 110.0 |
59 | India | 65.0 | 50.0 |
151 | Vatican | NaN | NaN |
45 | Finland | 80.0 | 80.0 |
88 | Malta | 60.0 | NaN |
12 | Belarus | 70.0 | 90.0 |
48 | Germany | 80.0 | 80.0 |
32 | Cuba | NaN | NaN |
46 | France | 110.0 | 130.0 |
119 | Russia | 90.0 | 90.0 |
148 | Uruguay | NaN | NaN |
25.3. Validate results#
Check if speed limit is too high
df_trucks.loc[
df_trucks.trucks_and_trailers > 200
]
country | trucks_and_trailers | trucks_and_trailers_non_built_up | |
---|---|---|---|
118 | Romania | 8090.0 | 90.0 |
Here is the original data
df.loc[
(
df_trucks.trucks_and_trailers > 200
)
]
country | Within towns(officially: within built-up area[4] or Urban road) | Automobiles & motorcycles (single carriageway) | Automobiles & motorcycles Expressways/motorways (dual carriageway) | trucks_and_trailers | trucks_and_trailers_non_built_up | Enforcement tolerance | Unnamed: 7 | |
---|---|---|---|---|---|---|---|---|
118 | Romania Main article: Speed limits in Romania | 5070 (some DN stretches) | 90100 (E-roads) | 130[fn 30] (motorways)100 (expressways) | 8090 (E-roads) | 90 (expressways)110 (motorways) | 10 km/h | NaN |
Other columns
df.loc[
(
df_trucks.trucks_and_trailers_non_built_up > 200
)
]
country | Within towns(officially: within built-up area[4] or Urban road) | Automobiles & motorcycles (single carriageway) | Automobiles & motorcycles Expressways/motorways (dual carriageway) | trucks_and_trailers | trucks_and_trailers_non_built_up | Enforcement tolerance | Unnamed: 7 |
---|
Fix the data
df_trucks.loc[df_trucks.country=="Romania", 'trucks_and_trailers'] = 90
/Users/lei.ma/opt/anaconda3/envs/mini-code/lib/python3.6/site-packages/pandas/core/indexing.py:965: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
self.obj[item] = s
Validate modifications
df_trucks.loc[
df_trucks.trucks_and_trailers > 200
]
country | trucks_and_trailers | trucks_and_trailers_non_built_up |
---|
Check if speed limti is too low
df_trucks.loc[
df_trucks.trucks_and_trailers < 50
]
country | trucks_and_trailers | trucks_and_trailers_non_built_up | |
---|---|---|---|
71 | Kiribati | 30.0 | 30.0 |
131 | Sri Lanka | 40.0 | 70.0 |
147 | United States | 16.0 | 129.0 |
df.loc[
(
df_trucks.trucks_and_trailers < 50
)
]
country | Within towns(officially: within built-up area[4] or Urban road) | Automobiles & motorcycles (single carriageway) | Automobiles & motorcycles Expressways/motorways (dual carriageway) | trucks_and_trailers | trucks_and_trailers_non_built_up | Enforcement tolerance | Unnamed: 7 | |
---|---|---|---|---|---|---|---|---|
71 | Kiribati[38] | 30 (church/school/bus stop zones; pedestrian c... | 60 | NaN | 30 | 30 | NaN | NaN |
131 | Sri Lanka | 50 (31 mph) | 70 (43 mph) | 70–100 (43–62 mph) (when 100 in expressways: p... | 40 (25 mph) (TukTuk) | 40–70 (25–43 mph) | NaN | NaN |
147 | United States[fn 1]Main articles: Speed limits... | 40–120 (25–75 mph)[citation needed] | 70-120 (45–75 mph)[fn 40] | 100–130 (60–80 mph)[fn 41] 137 (85 mph) is all... | Restrictions only in few states, typically 16 ... | 89–129 (55–80 mph)[fn 41] | States have jurisdiction over speed limits. En... | NaN |
df_trucks.loc[df_trucks.country=="United States", 'trucks_and_trailers'] = np.nan
/Users/lei.ma/opt/anaconda3/envs/mini-code/lib/python3.6/site-packages/pandas/core/indexing.py:965: SettingWithCopyWarning:
A value is trying to be set on a copy of a slice from a DataFrame.
Try using .loc[row_indexer,col_indexer] = value instead
See the caveats in the documentation: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html#returning-a-view-versus-a-copy
self.obj[item] = s
df_trucks.loc[
df_trucks.trucks_and_trailers < 50
]
country | trucks_and_trailers | trucks_and_trailers_non_built_up | |
---|---|---|---|
71 | Kiribati | 30.0 | 30.0 |
131 | Sri Lanka | 40.0 | 70.0 |
trucks_and_trailers_non_built_up column
df.loc[
(
df_trucks.trucks_and_trailers_non_built_up < 50
)
]
country | Within towns(officially: within built-up area[4] or Urban road) | Automobiles & motorcycles (single carriageway) | Automobiles & motorcycles Expressways/motorways (dual carriageway) | trucks_and_trailers | trucks_and_trailers_non_built_up | Enforcement tolerance | Unnamed: 7 | |
---|---|---|---|---|---|---|---|---|
71 | Kiribati[38] | 30 (church/school/bus stop zones; pedestrian c... | 60 | NaN | 30 | 30 | NaN | NaN |
25.4. Save Results#
df_trucks.to_csv("assets/export/road-speed-limits-by-country/speed_limits_by_countries.csv", index=False)
print(
df_trucks.fillna('-').to_markdown()
)
| | country | trucks_and_trailers | trucks_and_trailers_non_built_up |
|----:|:--------------------------------|:----------------------|:-----------------------------------|
| 0 | Åland (Finland) | - | - |
| 1 | Albania | 70.0 | 80.0 |
| 2 | Argentina | 80.0 | 80.0 |
| 3 | Armenia | 90.0 | 90.0 |
| 4 | Aruba | - | - |
| 5 | Andorra | - | - |
| 6 | Australia | 100.0 | 110.0 |
| 7 | Austria | 100.0 | 100.0 |
| 8 | Azerbaijan | - | - |
| 9 | Azores | - | 80.0 |
| 10 | Bahamas | - | - |
| 11 | Bahrain | - | - |
| 12 | Belarus | 70.0 | 90.0 |
| 13 | Belgium | 70.0 | 90.0 |
| 14 | Belgium: Wallonia Brussels | 90.0 | 90.0 |
| 15 | Belize | - | - |
| 16 | Benin | - | - |
| 17 | Bhutan | - | - |
| 18 | Bosnia and Herzegovina | 80.0 | - |
| 19 | Brazil | 90.0 | 90.0 |
| 20 | Brunei | 80.0 | 80.0 |
| 21 | Bulgaria | 70.0 | 100.0 |
| 22 | Cambodia | 80.0 | 100.0 |
| 23 | Cameroon | - | - |
| 24 | Canada | 120.0 | 130.0 |
| 25 | People's Republic of China | 80.0 | 100.0 |
| 26 | Chile | 100.0 | 100.0 |
| 27 | Christmas Island | - | 90.0 |
| 28 | Colombia | 60.0 | 90.0 |
| 29 | Costa Rica | 60.0 | 60.0 |
| 30 | Côte d'Ivoire | 90.0 | - |
| 31 | Croatia | 80.0 | 80.0 |
| 32 | Cuba | - | - |
| 33 | Curaçao | - | - |
| 34 | Cyprus | 80.0 | 100.0 |
| 35 | Czech Republic | 80.0 | 80.0 |
| 36 | Denmark | 80.0 | 80.0 |
| 37 | Dominican Republic | - | - |
| 38 | Ecuador | 70.0 | 90.0 |
| 39 | Egypt | - | - |
| 40 | El Salvador | - | - |
| 41 | Estonia | 90.0 | 90.0 |
| 42 | Ethiopia | - | - |
| 43 | Faroe Islands (Denmark) | - | - |
| 44 | Fiji | - | - |
| 45 | Finland | 80.0 | 80.0 |
| 46 | France | 110.0 | 130.0 |
| 47 | Georgia | 60.0 | 80.0 |
| 48 | Germany | 80.0 | 80.0 |
| 49 | Gibraltar (UK) | - | - |
| 50 | Greece | 80.0 | 80.0 |
| 51 | Greenland (Denmark) | - | - |
| 52 | Guernsey | - | 56.0 |
| 53 | Guyana | - | - |
| 54 | Haiti | - | - |
| 55 | Honduras | - | - |
| 56 | Hong Kong | 70.0 | 70.0 |
| 57 | Hungary | 70.0 | 80.0 |
| 58 | Iceland | 80.0 | 80.0 |
| 59 | India | 65.0 | 50.0 |
| 60 | Indonesia | 80.0 | 80.0 |
| 61 | Iran | 110.0 | 110.0 |
| 62 | Iraq | 90.0 | 120.0 |
| 63 | Ireland | 90.0 | 100.0 |
| 64 | Isle of Man | - | - |
| 65 | Israel | 80.0 | 90.0 |
| 66 | Italy | 70.0 | 80.0 |
| 67 | Jamaica | - | - |
| 68 | Japan | 80.0 | 80.0 |
| 69 | Jersey | - | 64.0 |
| 70 | Kazakhstan | - | - |
| 71 | Kiribati | 30.0 | 30.0 |
| 72 | Kosovo | - | - |
| 73 | Kuwait | 100.0 | 120.0 |
| 74 | Kyrgyzstan | 70.0 | 90.0 |
| 75 | Laos | 90.0 | 100.0 |
| 76 | Latvia | 80.0 | 90.0 |
| 77 | Lebanon | - | - |
| 78 | Liberia | - | - |
| 79 | Liechtenstein | 80.0 | - |
| 80 | Lithuania | 90.0 | 90.0 |
| 81 | Luxembourg | 90.0 | 90.0 |
| 82 | Libya | - | - |
| 83 | Macau | - | - |
| 84 | North Macedonia | 100.0 | - |
| 85 | Malawi | - | 80.0 |
| 86 | Malaysia | 70.0 | 90.0 |
| 87 | Mali | - | - |
| 88 | Malta | 60.0 | - |
| 89 | Mauritius | - | - |
| 90 | Moldova | 70.0 | - |
| 91 | Morocco | - | 100.0 |
| 92 | Monaco | - | - |
| 93 | Mongolia | - | - |
| 94 | Montenegro | - | - |
| 95 | Mexico | 95.0 | - |
| 96 | Namibia | - | - |
| 97 | Micronesia | - | - |
| 98 | Nepal | - | 110.0 |
| 99 | Netherlands | - | - |
| 100 | New Caledonia | - | - |
| 101 | New Zealand | 90.0 | 90.0 |
| 102 | Niue | - | - |
| 103 | Nicaragua | - | - |
| 104 | Nigeria | - | - |
| 105 | Norfolk Island | - | 50.0 |
| 106 | North Korea | - | - |
| 107 | Norway | 80.0 | 80.0 |
| 108 | Oman | 80.0 | - |
| 109 | Palestine | 60.0 | 60.0 |
| 110 | Panama | - | 100.0 |
| 111 | Papua New Guinea | - | - |
| 112 | Pakistan | 80.0 | 110.0 |
| 113 | Peru | 90.0 | 100.0 |
| 114 | Philippines | 80.0 | 60.0 |
| 115 | Poland | 70.0 | 80.0 |
| 116 | Portugal | 80.0 | 100.0 |
| 117 | Qatar | - | - |
| 118 | Romania | 90.0 | 90.0 |
| 119 | Russia | 90.0 | 90.0 |
| 120 | Samoa | - | - |
| 121 | San Marino | - | - |
| 122 | Serbia | 70.0 | 80.0 |
| 123 | Singapore | 60.0 | 60.0 |
| 124 | Slovakia | 90.0 | 90.0 |
| 125 | Slovenia | 80.0 | 80.0 |
| 126 | Saudi Arabia | 60.0 | 100.0 |
| 127 | Somalia | 80.0 | 100.0 |
| 128 | South Africa | 60.0 | 80.0 |
| 129 | South Korea (Republic of Korea) | 60.0 | 80.0 |
| 130 | Spain | 80.0 | 90.0 |
| 131 | Sri Lanka | 40.0 | 70.0 |
| 132 | Sudan | - | - |
| 133 | Suriname | - | 80.0 |
| 134 | Sweden | 90.0 | 80.0 |
| 135 | Switzerland | 80.0 | 80.0 |
| 136 | Taiwan (Republic of China) | 80.0 | 90.0 |
| 137 | Tanzania | 100.0 | - |
| 138 | Thailand | 80.0 | 100.0 |
| 139 | Trinidad and Tobago | 50.0 | 65.0 |
| 140 | Tunisia | - | - |
| 141 | Turkey | 80.0 | 90.0 |
| 142 | Turkmenistan | - | - |
| 143 | Uganda | - | - |
| 144 | Ukraine | 90.0 | 80.0 |
| 145 | United Arab Emirates | 80.0 | 80.0 |
| 146 | United Kingdom | 97.0 | 113.0 |
| 147 | United States | - | 129.0 |
| 148 | Uruguay | - | - |
| 149 | Uzbekistan | 70.0 | 90.0 |
| 150 | Vanuatu | - | - |
| 151 | Vatican | - | - |
| 152 | Venezuela | 60.0 | 120.0 |
| 153 | Vietnam | 70.0 | 70.0 |
| 154 | Yemen | - | - |
| 155 | Zambia | 75.0 | 70.0 |
| 156 | Zimbabwe | 80.0 | 80.0 |
25.5. Visualize#
import matplotlib.pyplot as plt
import seaborn as sns;sns.set()
fig, ax = plt.subplots(figsize=(10, 20))
vis_col = "trucks_and_trailers"
sns.barplot(
y="country",
x=vis_col,
data=df_trucks.sort_values(by=vis_col, ascending=False).dropna(subset=[vis_col]),
ax=ax
)
ax.set_title("Speed Limits for Trucks and Trailers")
Text(0.5, 1.0, 'Speed Limits for Trucks and Trailers')
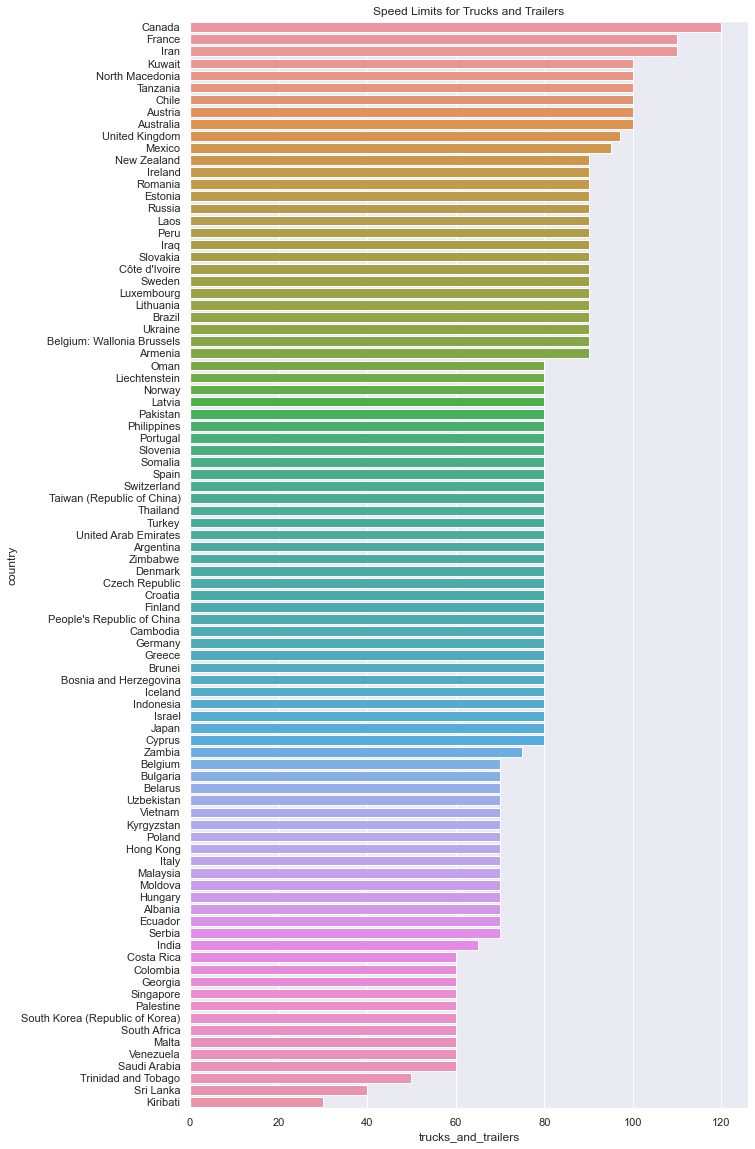
fig, ax = plt.subplots(figsize=(10, 20))
vis_col = "trucks_and_trailers_non_built_up"
sns.barplot(
y="country",
x=vis_col,
data=df_trucks.sort_values(by=vis_col, ascending=False).dropna(subset=[vis_col]),
ax=ax
)
#Trucks, or automobiles with trailer, outside built-up areas/highways
ax.set_title("Speed Limits for Trucks and Trailers outside Built-up Areas ")
Text(0.5, 1.0, 'Speed Limits for Trucks and Trailers outside Built-up Areas ')
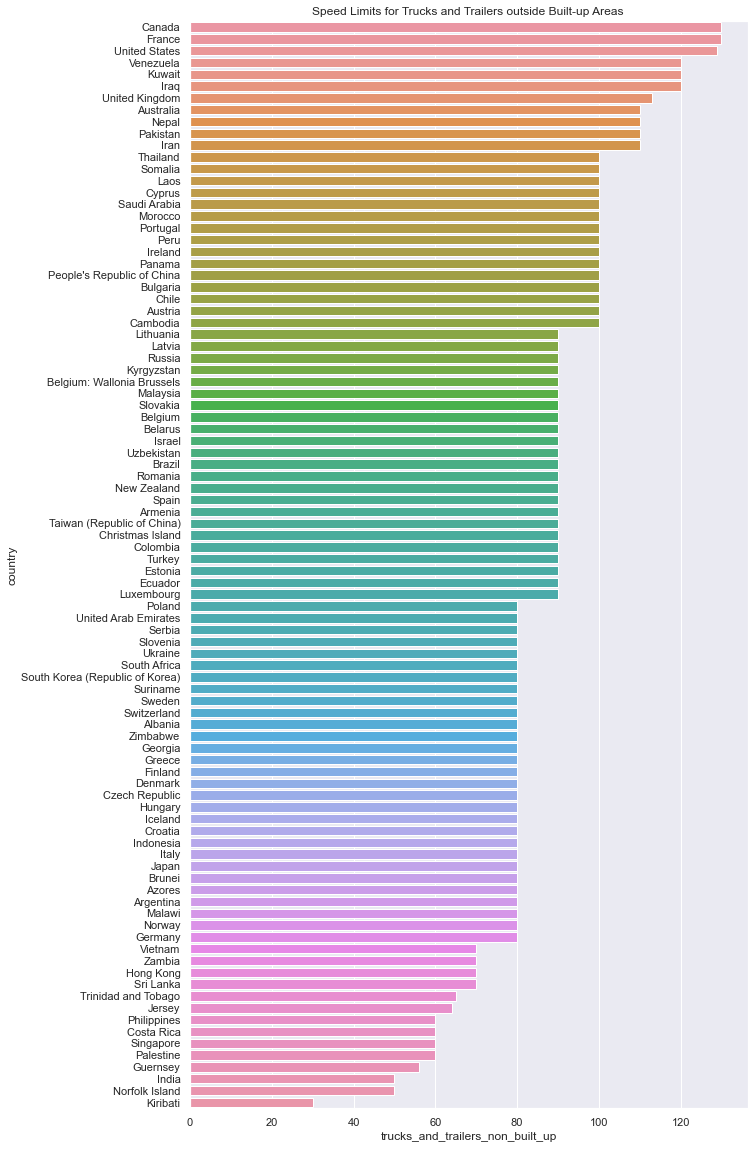